Smart Contracts Coding: Standards and Best Practises to Follow
Last Updated on: July 2, 2024
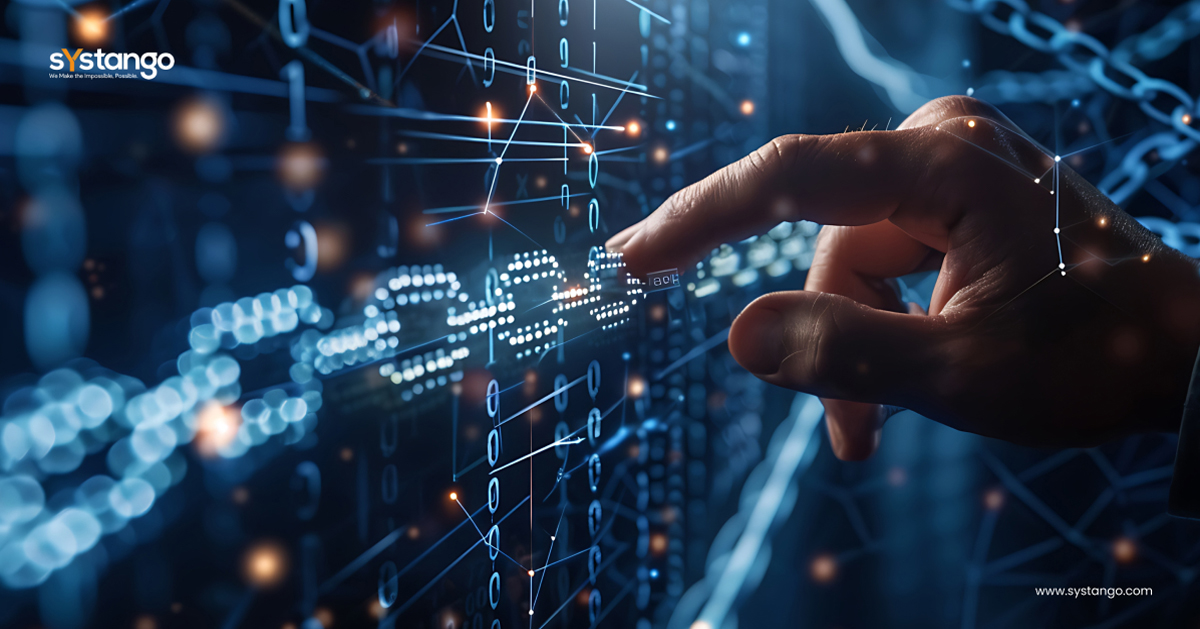
A smart contract is a digital agreement stored on a blockchain that automatically executes when predefined conditions are met. It eliminates the need for intermediaries and ensures transparent, secure, and efficient transactions. Smart contracts are immutable and decentralised, and enable trustless interactions between parties, enhancing the reliability of digital transactions.
When writing smart contract code, adhere to coding standards: consistent naming, indentation, and comments, plus modular and reusable code. Minimise complexity, loops, and errors, following security best practises like using the latest compiler, avoiding external calls, and employing modifiers and libraries.
Now, let’s explore the standards and best practises for secure and efficient smart contract coding in blockchain applications.
Key Takeaways on Smart Contracts Coding
Writing a Smart Contract Code – Best Practises
Let’s get started!
Writing a Smart Contract Code – Best Practises
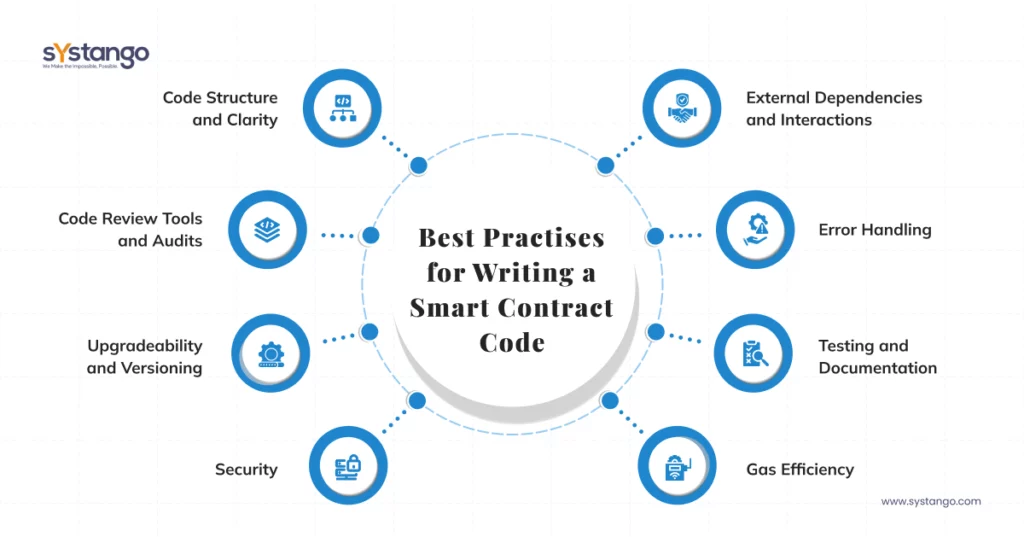
1. Security
- Check for potential vulnerabilities such as reentrancy, arithmetic overflow/underflow, and unhandled exceptions.
- Ensure that access controls and permissions are appropriately implemented.
- Verify that input validation and sanitisation are performed correctly.
- Review the usage of external contracts and libraries for potential security risks.
- Examples –
- Utilise mutex locks to prevent reentrancy attacks in smart contracts and implement checks for arithmetic overflow/underflow in financial calculations.
- Use role-based access control (RBAC) to limit administrative privileges.
- Assess third-party libraries for known vulnerabilities before integration and verify the audit history of external contracts before interacting with them.
2. Gas Efficiency
- Identify potential gas optimisations, such as reducing redundant calculations and minimising storage usage.
- Evaluate the use of data structures and algorithms to minimise gas consumption.
- Check for excessive loop iterations and recursion that could lead to high gas costs.
- Prepare a cost estimation sheet at the end of the development process to know the exact gas requirements for different functions.
- Examples –
- Implement binary search instead of linear search for gas-efficient data retrieval in sorted arrays or lists.
- Implement pagination for fetching large datasets to limit gas costs per transaction.
- Maintain a spreadsheet detailing gas costs for various contract functions and transactions to aid in budgeting and optimisation efforts.
3. Error Handling
- Ensure that errors and exceptions are handled appropriately to prevent unexpected behaviour.
- Verify that error messages are informative and helpful for debugging purposes.
- Review the usage of require, assert, and revert statements for proper error handling.
- Lock pragmas to a specific compiler version and use events to monitor contract activity.
- Examples –
- In a token contract, use requires statements to validate transfer conditions, such as checking for sufficient balance or preventing transfers to invalid addresses.
- Implement a try-catch block in a smart contract function that interacts with external contracts to handle potential exceptions and provide meaningful feedback to users.
- Utilise events to log important contract actions like token transfers or administrative changes, aiding in auditability and debugging processes.
- Avoid using block. number as a timestamp and avoid transfers to zero address.
Our Reading Recommendation: How is BaaS empowering Blockchain Developers?
4. Testing and Documentation
- Check for the presence of unit tests to validate the functionality and behaviour of the code.
- Evaluate the quality and coverage of the tests.
- Verify that the code includes sufficient documentation, including function and contract-level explanations and descriptions of important variables and events.
- The README of the project repository should be detailed with maximum information.
- Examples –
- Write unit tests to validate token transfer functionality, including edge cases like zero-value transfers or transfers exceeding the balance.
- Document each function’s purpose, parameters, return values, and potential exceptions using NatSpec comments or external documentation.
- In the README, outline deployment steps for deploying smart contracts to various networks, provide code snippets for interaction, and include troubleshooting tips for common deployment issues.
5. Code Structure and Clarity
- Ensure that the code is modular and follows a well-organised structure.
- Check if the code adheres to Solidity style guide conventions (e.g., using proper naming conventions, consistent indentation, and commenting).
- Verify the code is readable and understandable, with a clear function and variable names.
- Code should follow the NatSpec comments format to provide rich documentation for functions, return variables and more.
- Examples –
- Lock pragmas to specific compiler versions.
- Use commenting structure like –
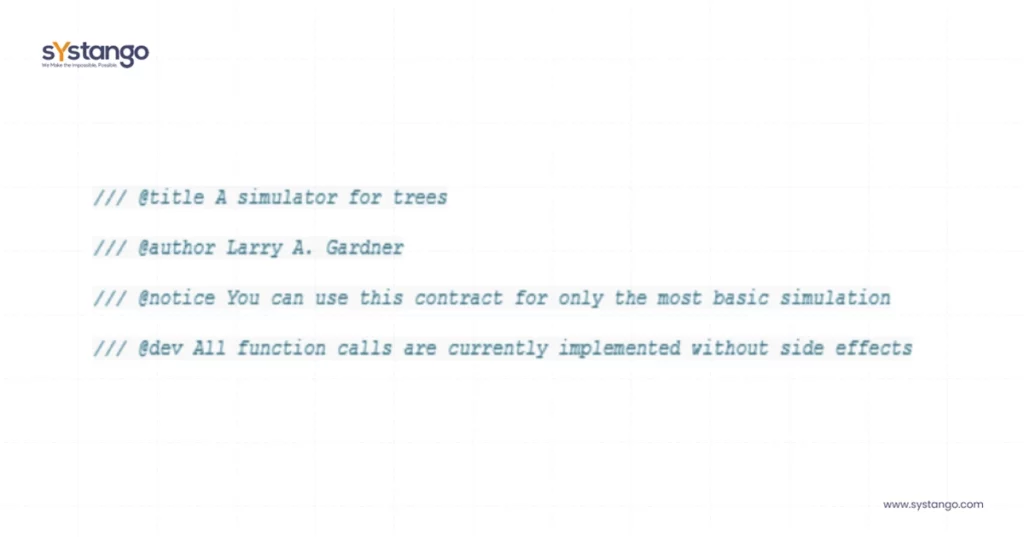
6. External Dependencies and Interactions
- Review any external contracts or libraries used and assess their security and reliability.
- Verify the correct implementation of interactions with external contracts, including handling return values, error cases, and gas considerations.
- Evaluate the trustworthiness of any external oracles, if used.
- Examples –
- Implement fallback functions or error handling mechanisms to gracefully handle failed interactions with external contracts, preventing contract halts or unexpected behaviour.
- Use decentralised oracle networks like Chainlink to source real-world data securely and reliably for smart contract applications, minimising centralised trust dependencies.
7. Upgradeability and Versioning
- If the code supports upgradeability, review the upgrade mechanism for potential security risks and impact on existing data and functionality.
- Check for proper versioning and deprecation mechanisms.
- Examples –
- Implement access control mechanisms and multisignature requirements for upgrade functions to prevent unauthorised modifications in upgradeable contracts.
- Use semantic versioning to indicate breaking changes, new features, and bug fixes in smart contract releases, facilitating version management and dependency tracking.
8. Code Review Tools and Audits
- Consider using automated code analysis tools and security scanners specifically designed for Solidity code.
- Use tools like Slither to publish the audit reports and bind them to
- Consider conducting a formal security audit by an external auditing firm.
- Example –
- Include Slither audit reports in project repositories or documentation to highlight identified issues and their resolution status, fostering trust and accountability.
Conclusion
Writing secure and efficient smart contract code is crucial for ensuring trust and reliability in blockchain applications. Follow these key practises:
- Prioritise security by mitigating vulnerabilities and implementing robust access controls.
- Optimise gas efficiency to minimise transaction costs.
- Handle errors effectively to ensure predictable behaviour.
- Maintain thorough testing and documentation.
- Structure code clearly and adhere to standards.
- Evaluate external dependencies for reliability.
- Plan for upgradeability and proper versioning.
- Utilise code review tools and conduct formal audits.
By adhering to these best practises, developers can build smart contracts that are resilient, transparent, and trustworthy, laying a solid foundation for blockchain-based applications.
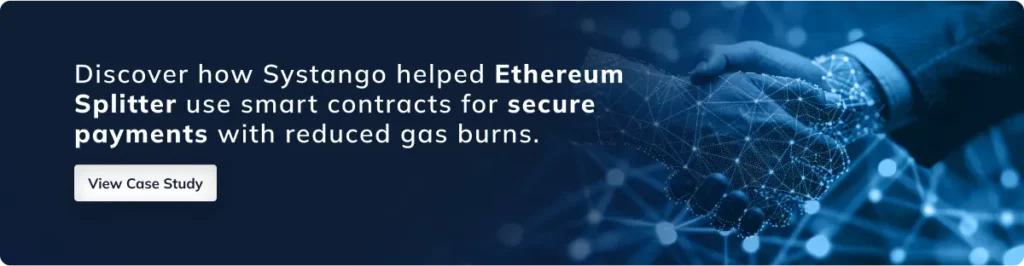